일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 | 31 |
- CSS
- media query
- 반응형 페이지
- 프론트엔드
- node.js
- github
- coding
- JS
- 그럼에도 불구하고
- max-width
- 변수
- Servlet
- 코딩테스트
- 자바문제풀이
- JavaScript
- java
- react
- frontend
- TypeScript
- webpack
- git
- @media
- react-router-dom
- node
- 코드업
- cleancode
- HTML
- 그럼에도불구하고
- redux
- 자바
- Today
- Total
그럼에도 불구하고
[Node.js] 애플리케이션 레벨 미들웨어 본문
[ 애플리케이션 레벨 미들웨어 ]
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 | const express = require('express'); const app = express(); app.use((req, res, next) => { console.log('In the middleware!'); next(); // Allows the request to continue to the next middleware in line }); app.use((req, res, next) => { console.log('In another middleware!'); res.send('<h1>Hello from Express!</h1>'); }); app.listen(3000); | cs |
const http = require('http');
const server = http.createServer(app);
server.listen(3000);
하지만 express와 그 안의 middleware 함수를 이용하면 아래와 같이 작성해도 위와 동일한 기능을 한다.
app.listen(3000);
http 선언을 하지 않아도 되며, sever 생성이 app.listen(3000);으로 끝난다.
nodemon을 통해 서버를 올리고 localhost:3000을 웹 페이지에 입력하면 아래와 같은 창이 나오게 된다.
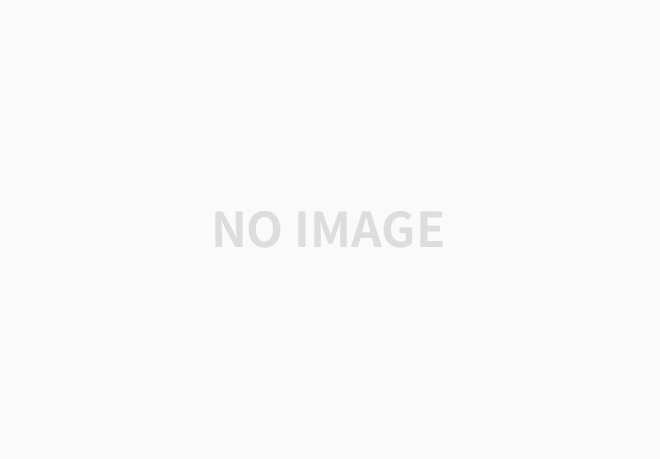
이번에는 들어오는 요청을 실제로 어떻게 처리하고 데이터를 추출하는지 알아보자
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 | const express = require('express'); const bodyParser = require('body-parser'); app.use(bodyParser.urlencoded({extended: false})); // 미들웨어 등록 app.use('/add-product', (req, res, next) => { res.send('<form action="/product" method="POST"><input type="text" name = "title"><button type ="submit">Add Product</button></form>'); }); app.use('/product', (req, res, next) => { console.log(req.body); res.redirect('/'); }) app.use('/', (req, res, next) => { res.send('<h1>Hello from Express!</h1>'); }); app.listen(3000); | cs |
add-product라는 곳에서 폼(form)이 있는 html 페이지를 반환하고 싶다고 가정해 보자.
위의 소스코드처럼 폼을 작성하고 text란에 내용을 적고 버튼을 누르면 /product 페이지로 넘어가게 되고, /product 페이지에서는 res.redirect('/');을 통해 다시 localhost:3000 페이지로 돌아오게 된다.
이때 form안의 text에 내용을 입력하고 버튼을 누르면 콘솔로 그 내용을 가져오게 작성하였지만 원래는 undefined가 나오게 된다.
( 위의 코드는 body-parser 때문에 제대로 된 값이 나옴 )
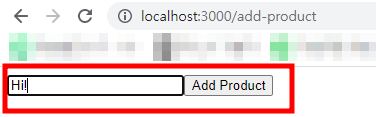
아래와 같이 console.log(req.body)가 undefined를 출력한다.

이는 req.가 이 본문에 편의 속성을 주는 것은 맞지만 기본적으로 req. 는 들어오는 요청 본문을 분석하려 하지 않기 때문에 분석해줄 수 있는 또 다른 미들웨어를 추가해서 구현해야 한다.
$ npm install --save body-parser
터미널에 위와 같이 입력하고 아래외 같이 작성해 주면 req.body가 제대로 된 값을 가져오게 된다.
const bodyParser = require('body-parser');
app.use(bodyParser.urlencoded({extended:false}); // 미들웨어 등록 { 키 : 값 }

'Node.js > Node.js basics' 카테고리의 다른 글
[Node.js] 오류 페이지 추가하기 (0) | 2023.02.02 |
---|---|
[Node.js] Router 사용하기 (0) | 2023.02.02 |
[Node.js] 미들웨어란? (0) | 2023.02.01 |
[Node.js] Express란? (1) | 2023.02.01 |
[Node.js] Nodemon 설치하기 (1) | 2023.01.31 |